Top 10 Python Interview Questions For Freshers
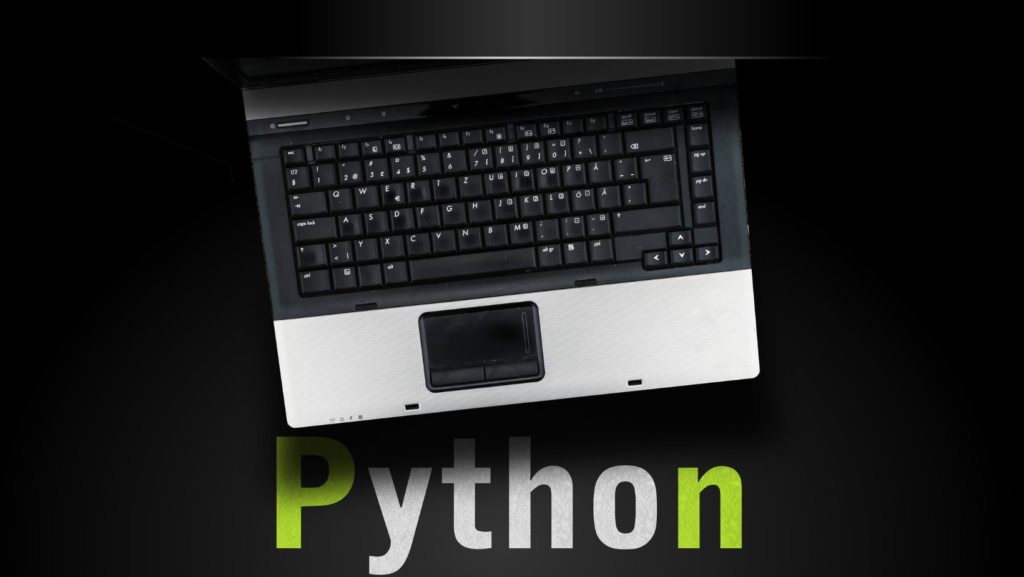
Table Content
Table of Contents
Introduction
In today’s highly competitive job market, it’s crucial to be well-prepared for interviews, especially when it comes to programming positions. Python, being one of the most popular programming languages, is frequently tested in interviews. To help you succeed in your Python interview, this comprehensive guide will cover the most common Python interview questions and provide detailed answers. By the end of this guide, you’ll have a solid understanding of these concepts and be fully prepared to impress your interviewer.
Python Basics
1. What is Python?
Python is a high-level programming language known for its clear and readable syntax. It was created by Guido van Rossum and first released in 1991. Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming. It is widely used for web development, data analysis, artificial intelligence, and more.
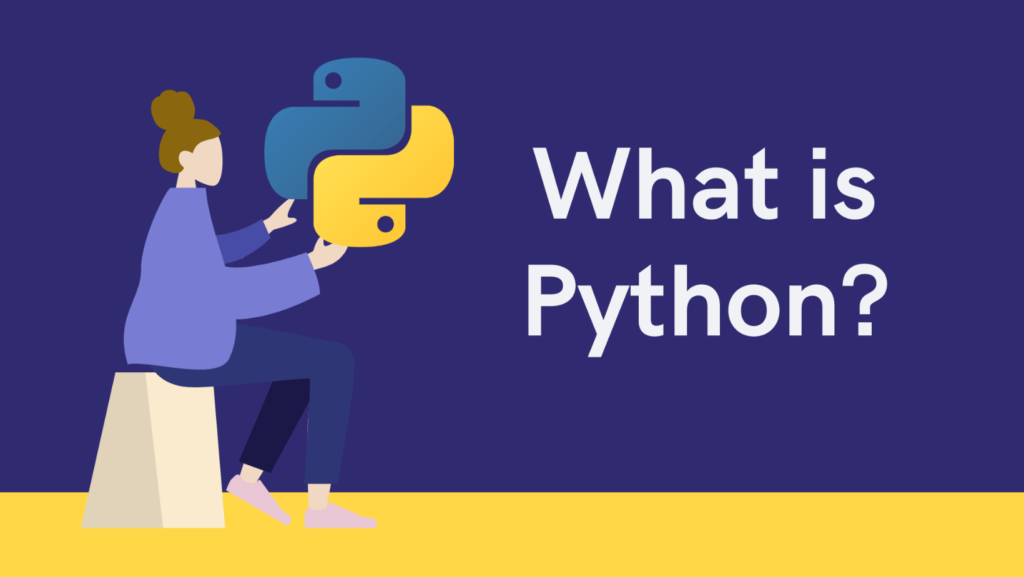
2. How does Python handle memory management?
Python uses automatic memory management, which means developers don’t need to explicitly allocate or deallocate memory. Python achieves this through a garbage collector that automatically reclaims memory when objects are no longer needed. In addition to garbage collection, Python also uses reference counting and cycle detection to effectively manage memory and prevent memory leaks.
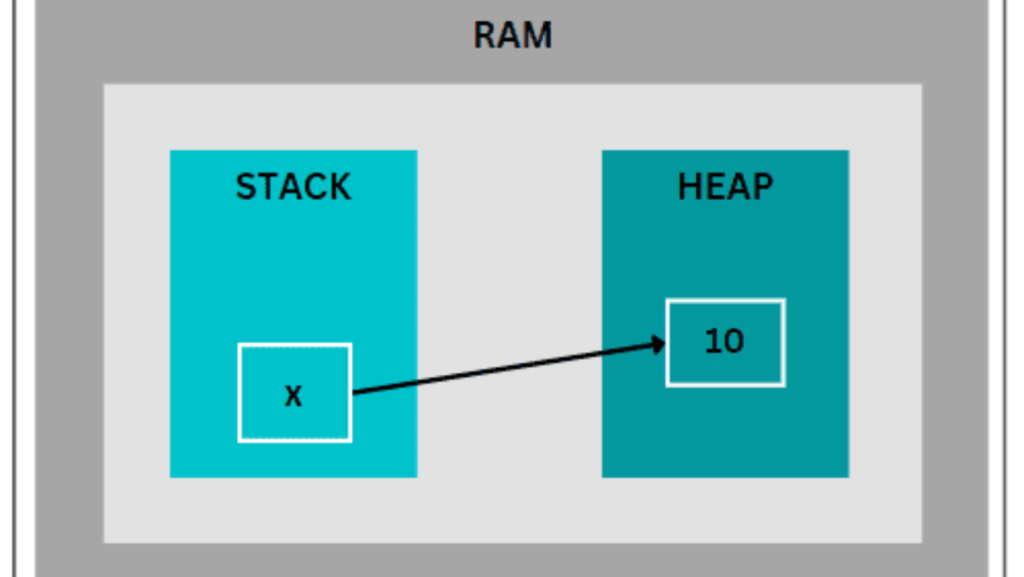
3. What are the different data types in Python?
In Python, the main data types include:
- Integer (int): Whole numbers without decimals.
- Floating-point (float): Numbers with decimals.
- String (str): Sequence of characters.
- Boolean (bool): Represents True or False.
- List: Ordered, mutable collections of items.
- Tuple: Ordered, immutable collections.
- Dictionary (dict): Key-value pairs.
- Set: Unordered, unique collections.
These data types cover a wide range of applications and are fundamental to Python programming.
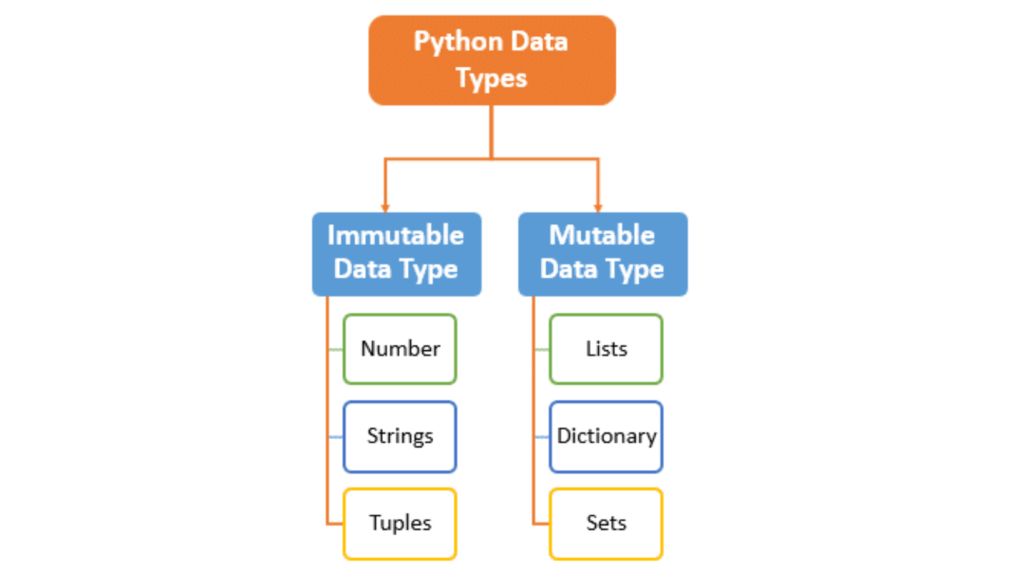
4. Explain the difference between a list and a tuple in Python\.
In Python, a list is a mutable data structure that can be modified after creation. It is denoted by square brackets [ ] and allows for adding, removing, or changing elements. On the other hand, a tuple is an immutable data structure denoted by parentheses ( ). Once created, a tuple cannot be modified. Tuples are often used for storing related pieces of data, such as coordinates or database records, while lists are more suitable for dynamic data manipulation.
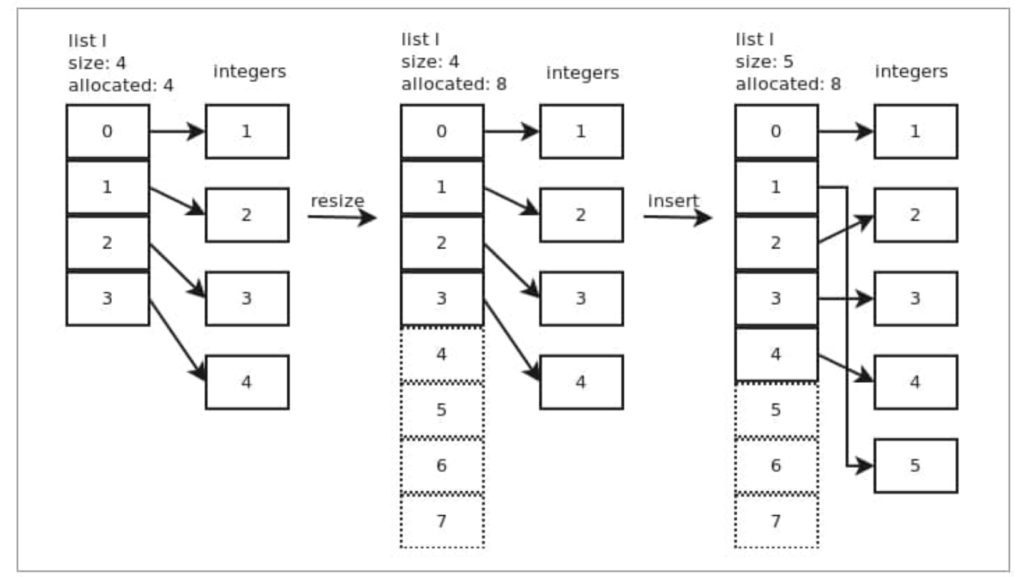
5. How do you handle file operations in Python?
In Python, you can handle file operations using the built-in open() function to open files in different modes (read, write, append, etc.). After opening a file, you can perform various operations like reading from it, writing to it, or manipulating its contents. It’s important to close the file using the close() method to release system resources once you’re done with it. Alternatively, you can use the with statement, which automatically closes the file when you’re done with it.
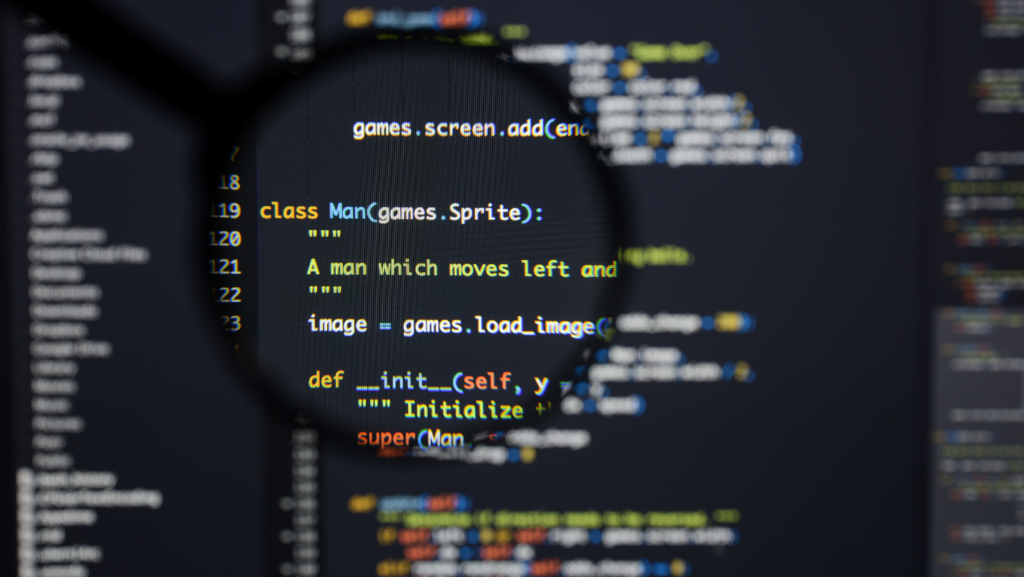
Object-Oriented Programming (OOP) in Python
1. What are the principles of OOP?
Object-Oriented Programming (OOP) is a programming paradigm that focuses on objects and their interactions. In Python, the principles of OOP include encapsulation, inheritance, and polymorphism. Encapsulation refers to the bundling of data and methods into a single unit called a class. Inheritance allows classes to inherit properties and behaviors from other classes. Polymorphism enables objects of different classes to be used interchangeably based on a common interface.
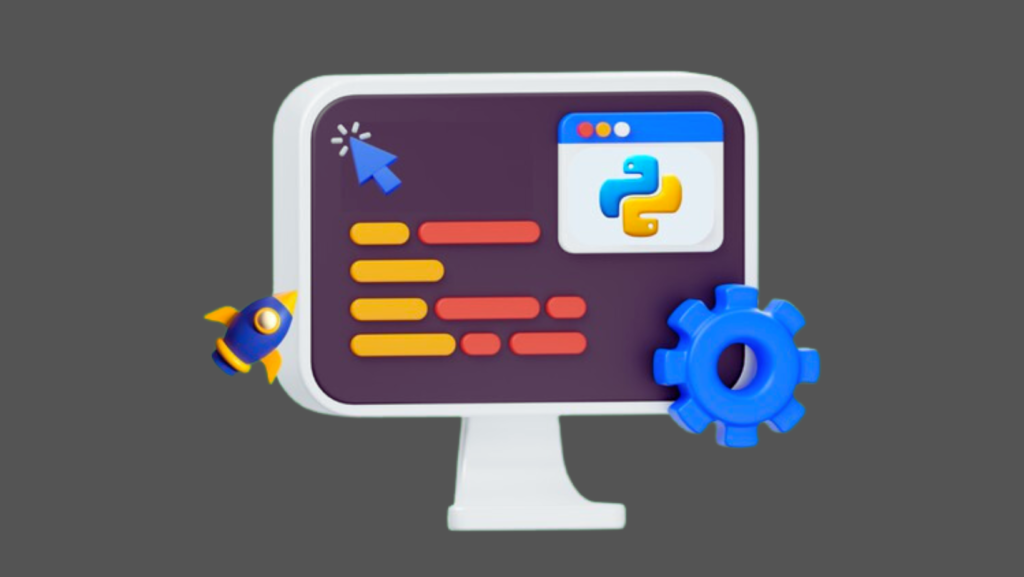
2. How do you define a class in Python?
To define a class in Python, you use the `class` keyword followed by the class name. Within the class, you can define class attributes (variables shared by all instances of the class) and methods (functions associated with the class). The class provides a blueprint for creating objects (instances) that share the same structure and behavior defined in the class.
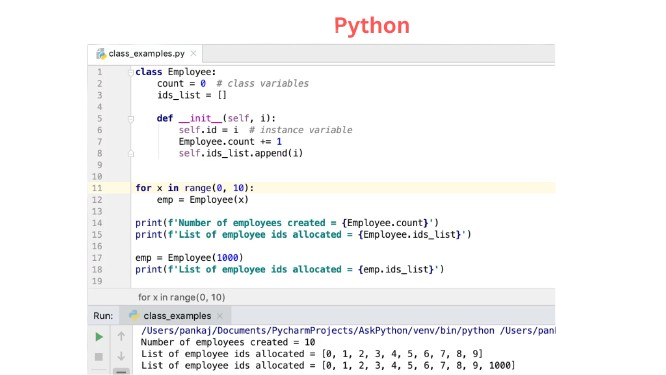
3. What is the difference between an instance method and a class method?
In Python, instance methods operate on an instance of a class. They have access to instance-specific data and can modify it. Instance methods are defined using the `self` parameter, which refers to the instance itself. On the other hand, class methods operate on the class itself rather than specific instances. They are defined using the `@classmethod` decorator and have access to class-level data. Class methods are useful when you want to perform operations that affect the entire class rather than individual instances.
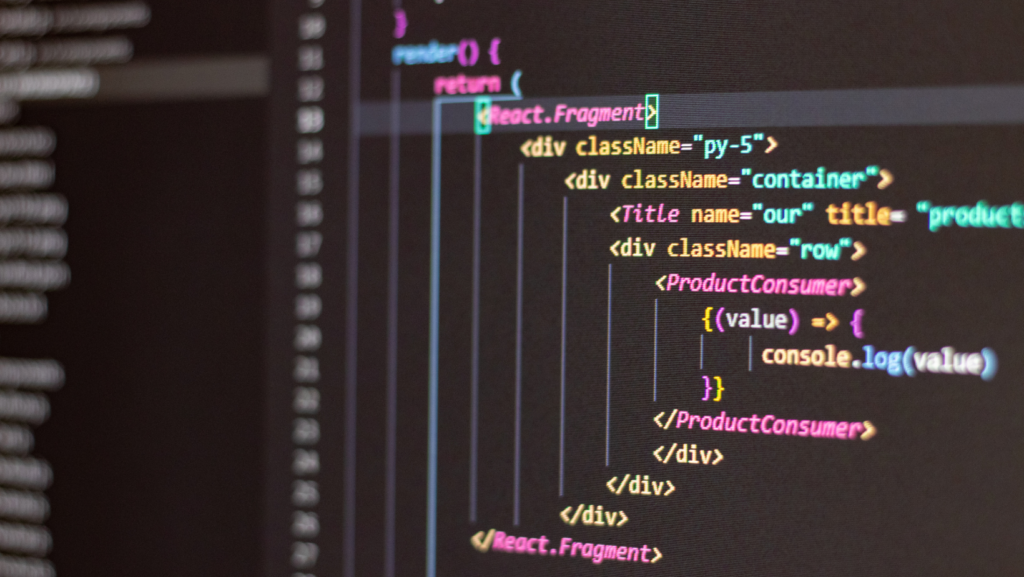
4. How does Python handle multithreading?
In Python, multithreading is handled primarily through the built-in threading module. However, due to the Global Interpreter Lock (GIL), which allows only one thread to execute Python bytecode at a time, multithreading in Python is more suitable for I/O-bound tasks rather than CPU-bound tasks.
Python’s threading module provides a high-level interface for creating, starting, and managing threads. It also offers synchronization primitives like locks and semaphores to coordinate access to shared resources among threads. For CPU-bound tasks, Python’s multiprocessing module is often preferred, as it bypasses the GIL by spawning separate processes instead of threads.
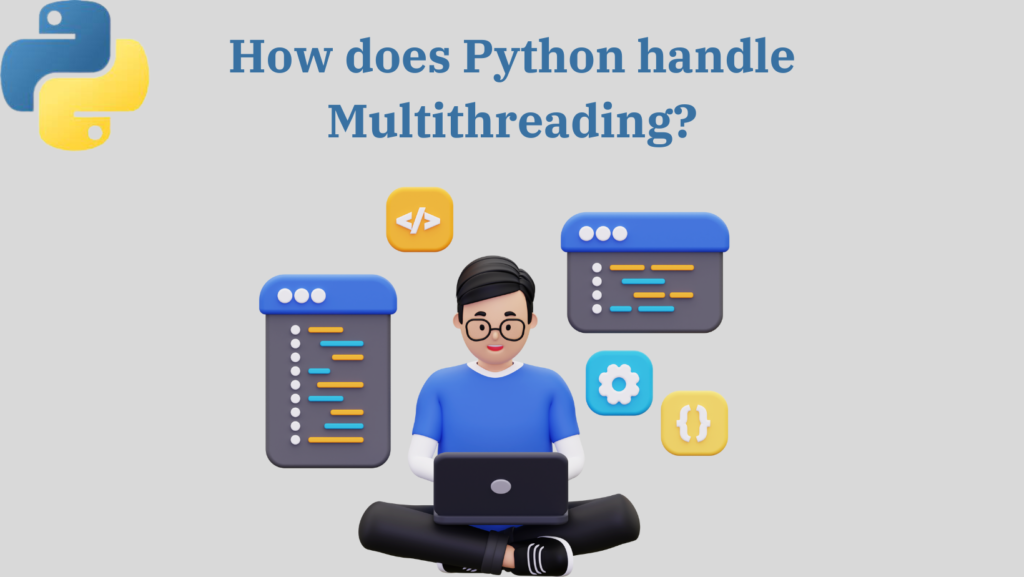
Python Data Structures and Algorithms
1. What is the difference between a set and a frozenset in Python?
In Python, a set is a mutable collection of unique elements, while a frozenset is an immutable version of a set. Sets are denoted by curly braces { }, and frozensets are created using the `frozenset()` function. One key difference is that sets can be modified, allowing for adding or removing elements, while frozensets cannot be changed once created. Another difference is that frozensets can be used as keys in dictionaries, while sets cannot.
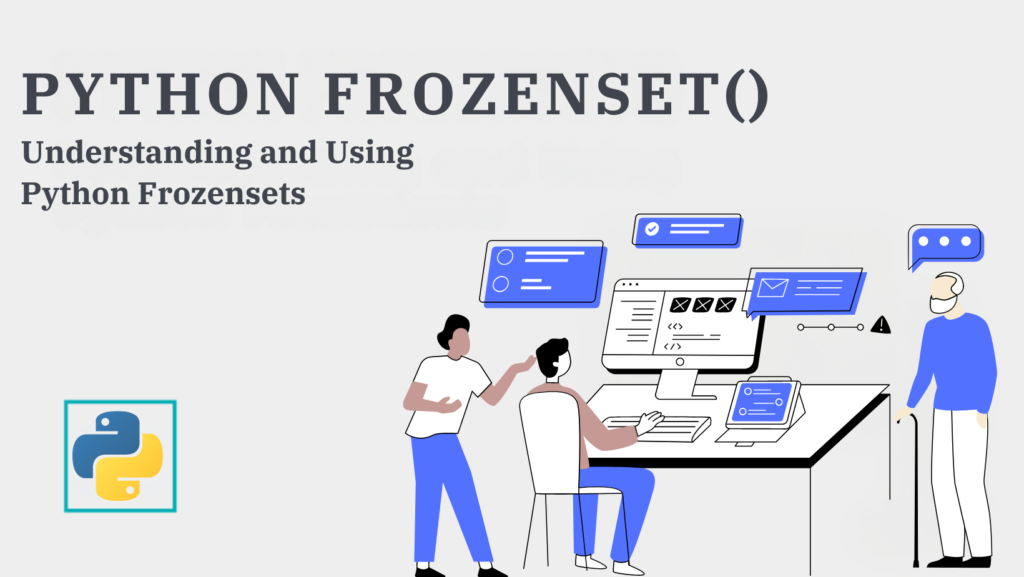
2. Explain the concept of recursion in Python
Recursion is a programming technique where a function calls itself to solve a problem. The function breaks down a complex problem into smaller, more manageable subproblems until a base case is reached. Each recursive call works on a smaller input, and the results are combined to solve the original problem. Recursion is commonly used in solving problems with repetitive structures, such as traversing trees or calculating factorials.
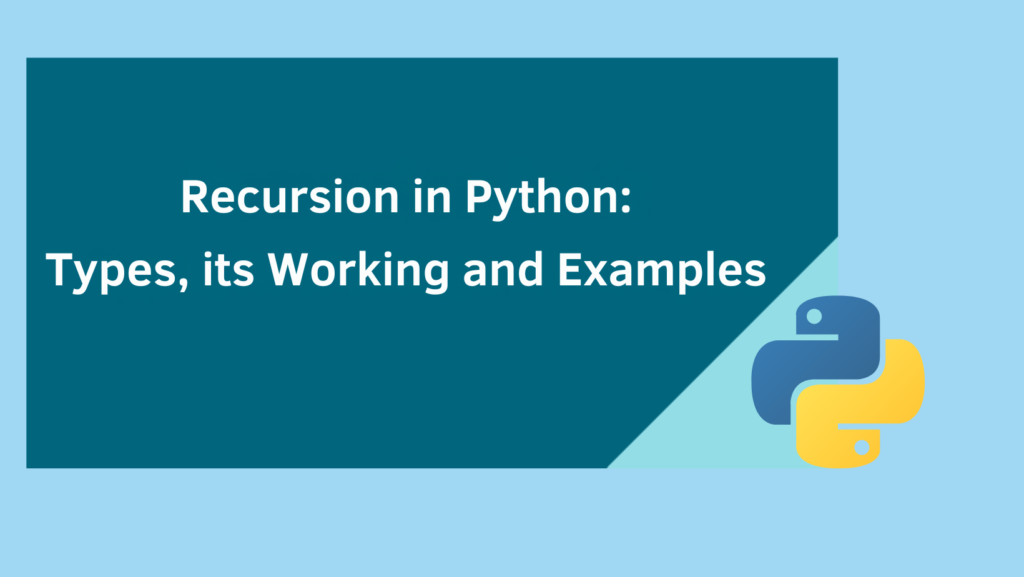
3. How do you sort a list of objects in Python?
To sort a list of objects in Python, you can use the `sorted()` function with a custom key parameter. The key parameter specifies a function that takes an element from the list and returns a value to use for sorting. Alternatively, you can implement the `__lt__()` method in the object class. This method defines the less than (<) comparison operator and allows for custom sorting based on object attributes.
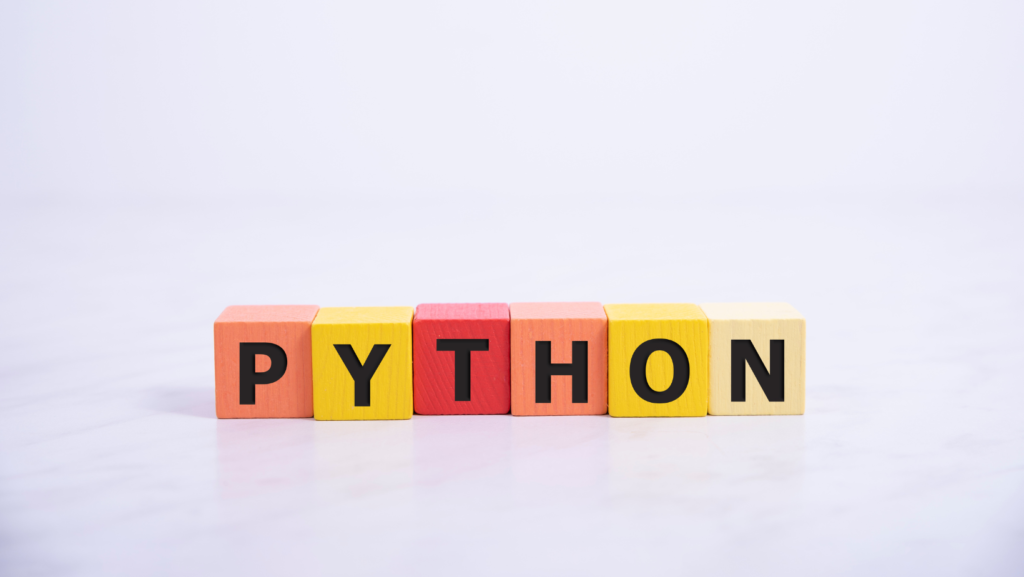
4. What is the difference between the "==" operator and the "is" keyword in Python?
The “==” operator in Python is used to compare the values of two objects to check if they are equal. It compares the contents of the objects to determine equality. On the other hand, the “is” keyword is used to check if two variables refer to the same object in memory, i.e., if they have the same identity. While “==” compares values, “is” compares identities
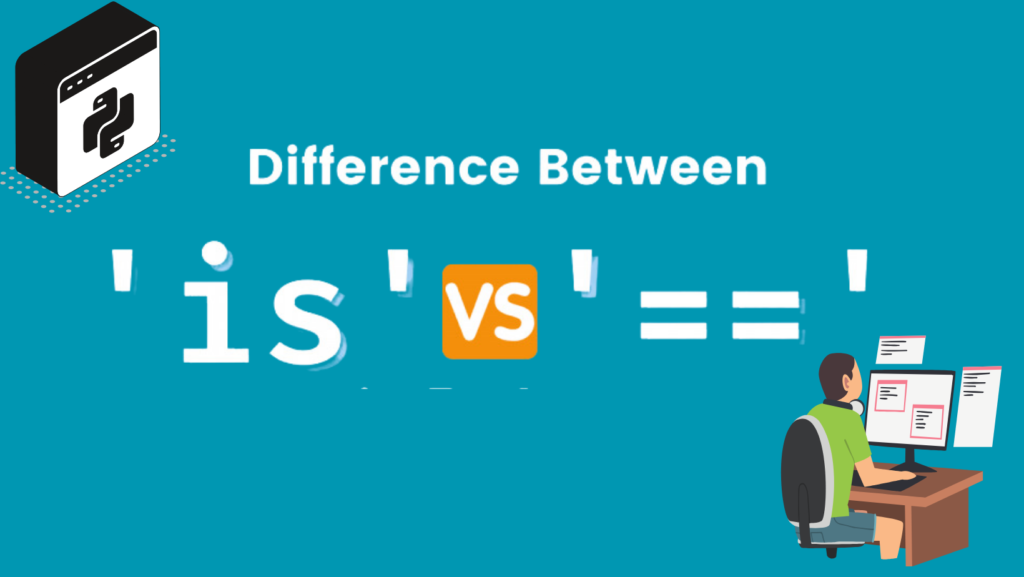
Libraries and Packages in Python
1. What is NumPy and why is it important in Python?
NumPy is a powerful library for numerical computing in Python. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays efficiently. NumPy is widely used in scientific computing, data analysis, and machine learning. Its array operations are significantly faster than traditional Python lists, making it a crucial tool for handling large datasets.
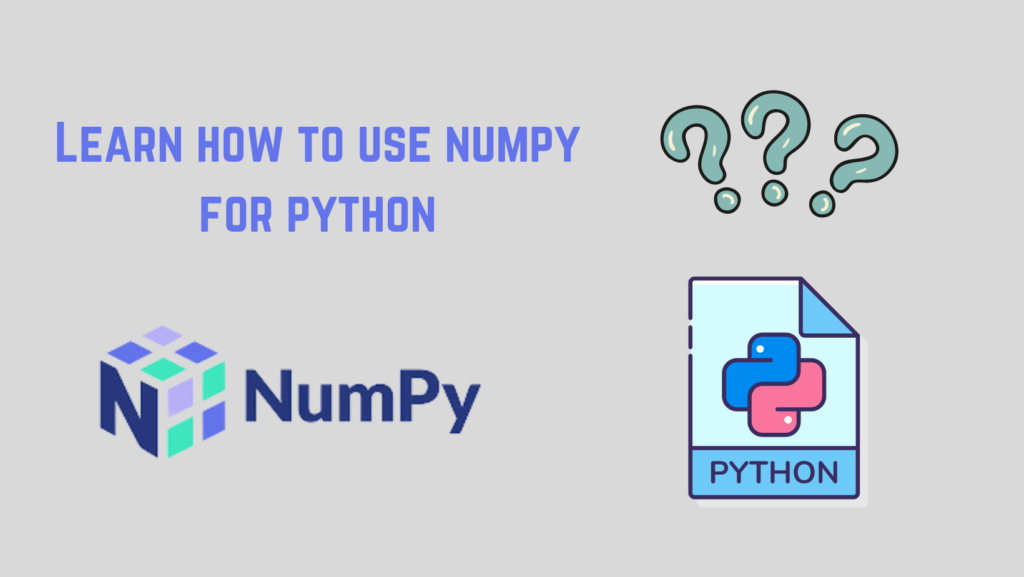
2. What are decorators in Python?
Decorators are a Python feature that allows you to modify the behavior of functions or classes without modifying their source code. Decorators are indicated by the `@` symbol followed by the decorator name, placed above the function or class definition. They provide a way to add functionality to existing code, such as logging, timing, or access control, by wrapping the original code with additional code. Decorators are a powerful tool for code reuse and enhancing the functionality of Python applications.
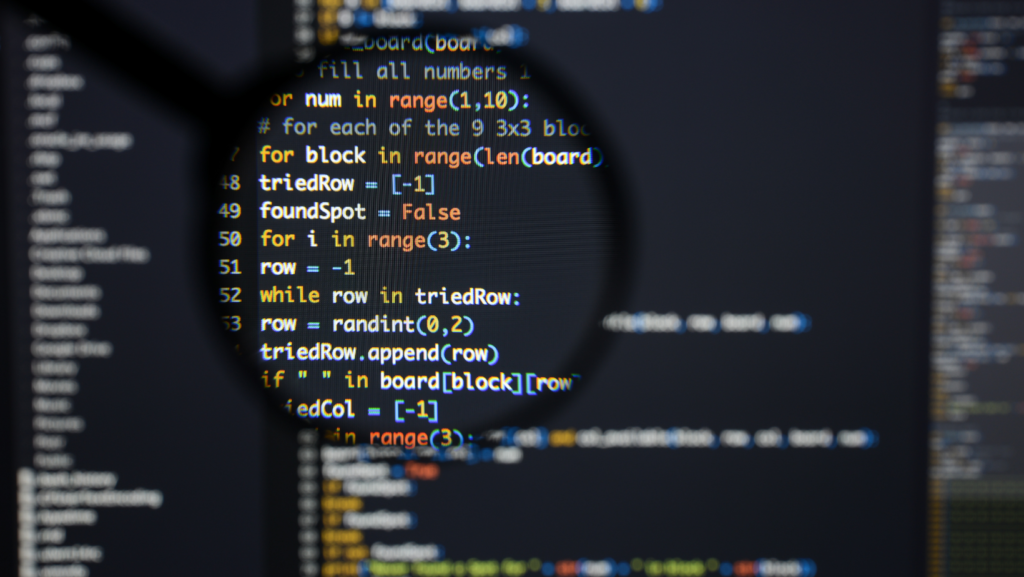
3. What is the purpose of the "os" module in Python?
The “os” module in Python provides a way to use operating system-dependent functionality, such as interacting with the file system, managing processes, and executing system commands. It offers various functions and constants that abstract the underlying operating system and provide a consistent interface for platform-specific operations. The “os” module is essential for creating cross-platform applications and automating system-related tasks.
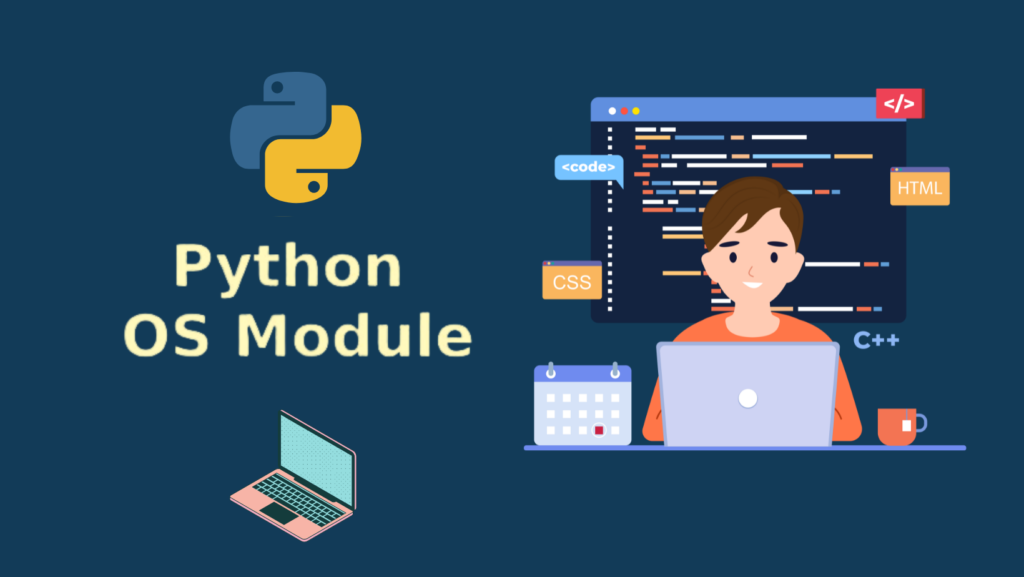
4. What is the purpose of the "lambda" keyword in Python?
The “lambda” keyword in Python is used to create anonymous functions, also known as lambda functions. These are small, inline functions that can have any number of arguments but can only have one expression. Lambda functions are commonly used when you need a simple function for a short period of time, often as an argument to higher-order functions like map(), filter(), or sorted().
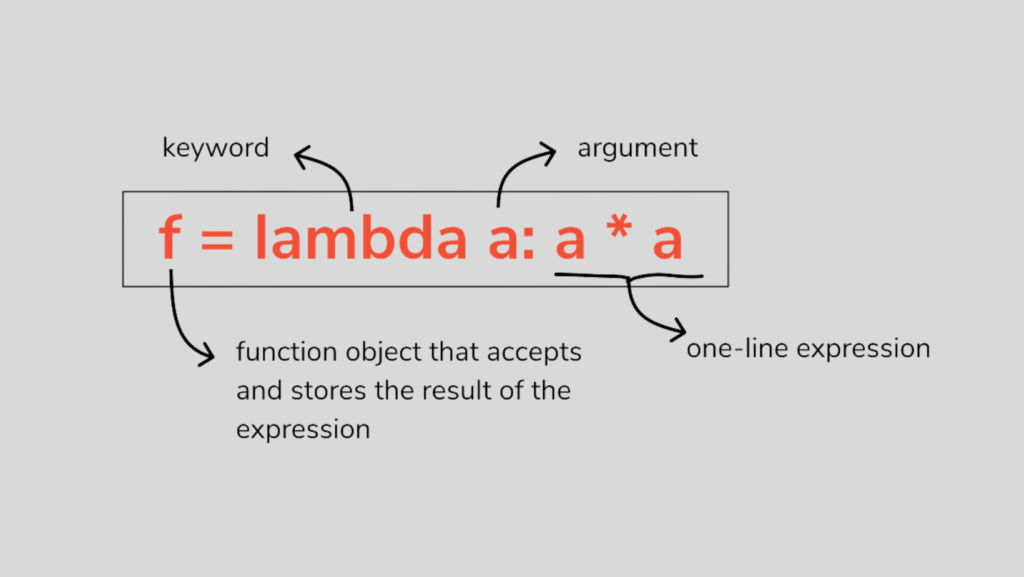
Why Should One Consider Enrolling In Anubhav For Python Training?
Python is one of the most popular programming languages and has gained immense popularity over the years. As a result, the demand for skilled Python developers has increased significantly. If you are considering a career in Python development or want to enhance your existing skills, enrolling in Anubhav for Python training can be highly beneficial. In this blog post, we will discuss the reasons why Anubhav stands out and why it is a great choice for Python training.
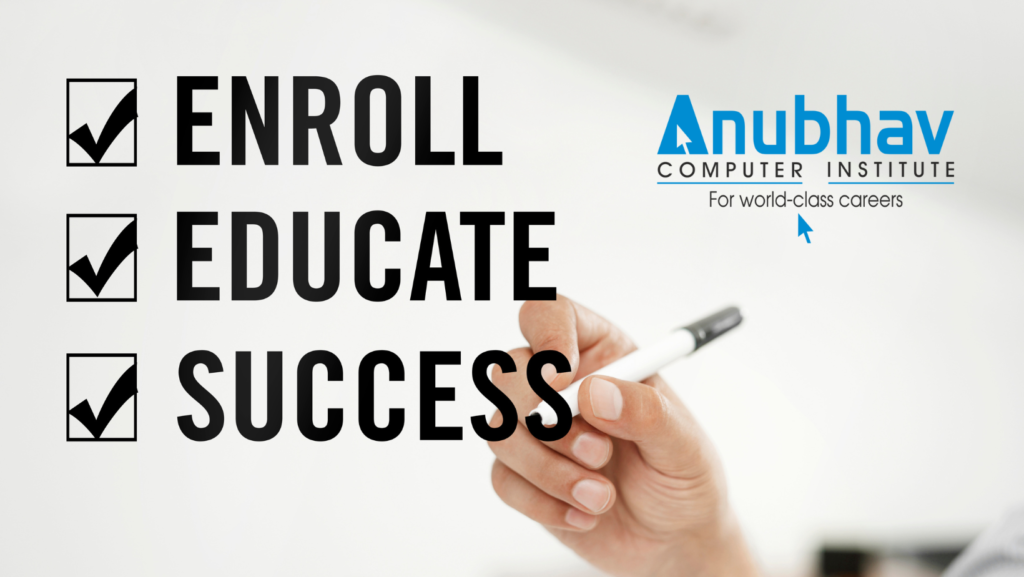
1. Expert Instructors
Anubhav takes pride in its team of expert instructors who have years of experience in the industry. They not only possess a deep understanding of the Python programming language but also have hands-on experience working on real-world projects. The instructors at Anubhav ensure that the training is comprehensive, up-to-date, and relevant to the latest industry trends. Their expertise enables them to answer complex questions and provide practical insights that go beyond theoretical knowledge.
2. Hands\-on Learning
Anubhav believes in the power of hands-on learning and provides ample opportunities for students to apply their knowledge in practical scenarios. The training includes coding exercises, projects, and real-world examples that allow students to practice and implement what they have learned. This interactive learning approach not only helps in reinforcing the concepts but also enhances problem-solving skills and boosts confidence.
3. Industry Connections and Networking
Anubhav understands the importance of networking and building industry connections for career growth. As part of the Python training program, students get the opportunity to interact with guest speakers, industry experts, and fellow Python enthusiasts. This enables them to expand their professional network, learn from experienced professionals, and gain insights into the industry best practices. Anubhav also provides job placement assistance to help students kickstart their careers in Python.
4. Affordable Pricing and Value for Money
One of the key advantages of choosing Anubhav for Python training is its affordable pricing. Despite providing top-quality training and a comprehensive curriculum, Anubhav ensures that the courses are reasonably priced, making it accessible to a wide range of learners. Students get excellent value for their investment as they gain in-demand skills and knowledge that can open up lucrative career opportunities in the Python ecosystem.
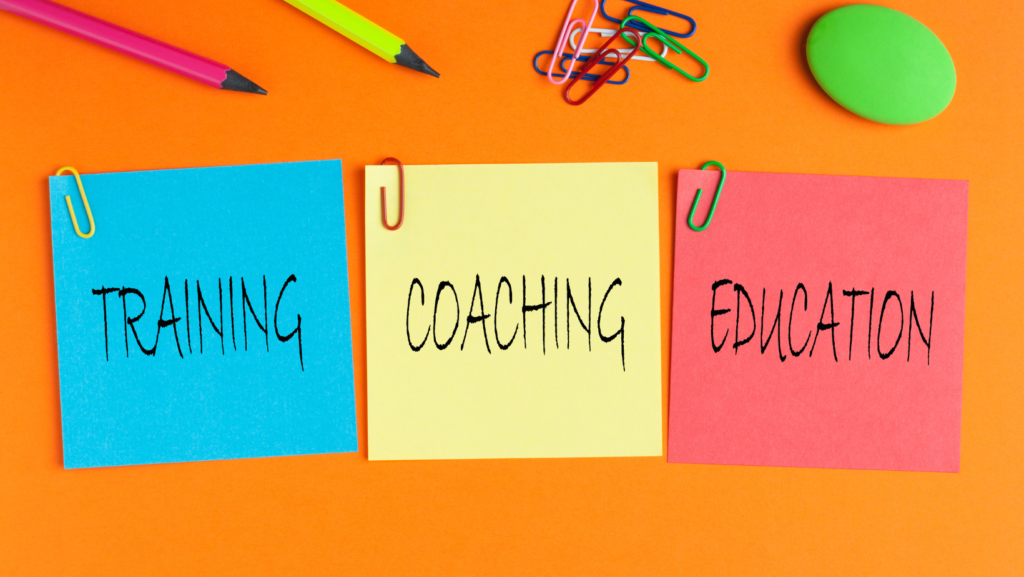
In conclusion, enrolling in Anubhav for Python training is a wise choice for anyone looking to kickstart or advance their career in Python development. With expert instructors, a comprehensive curriculum, hands-on learning, industry connections, flexibility, and affordable pricing, Anubhav provides the perfect platform to acquire in-demand Python skills. Make a smart decision today and unlock your potential with Anubhav’s Python training program.
Remember, learning Python is not just about obtaining a certificate, but about gaining practical skills and knowledge that can catapult your career to new heights. So, what are you waiting for? Enroll in Anubhav’s Python training program and embark on an exciting journey towards becoming a proficient Python developer.
“Invest in your Python skills today and reap the rewards of a successful career tomorrow.”
Summary
This comprehensive guide has covered the most common Python interview questions, ranging from the basics of the language to advanced topics like object-oriented programming, data structures, and libraries. By mastering these concepts, you’ll be well-prepared for any Python interview. Remember to practice implementing these concepts in real-world scenarios to solidify your understanding. Thorough preparation and a strong foundation in Python will greatly increase your chances of success.
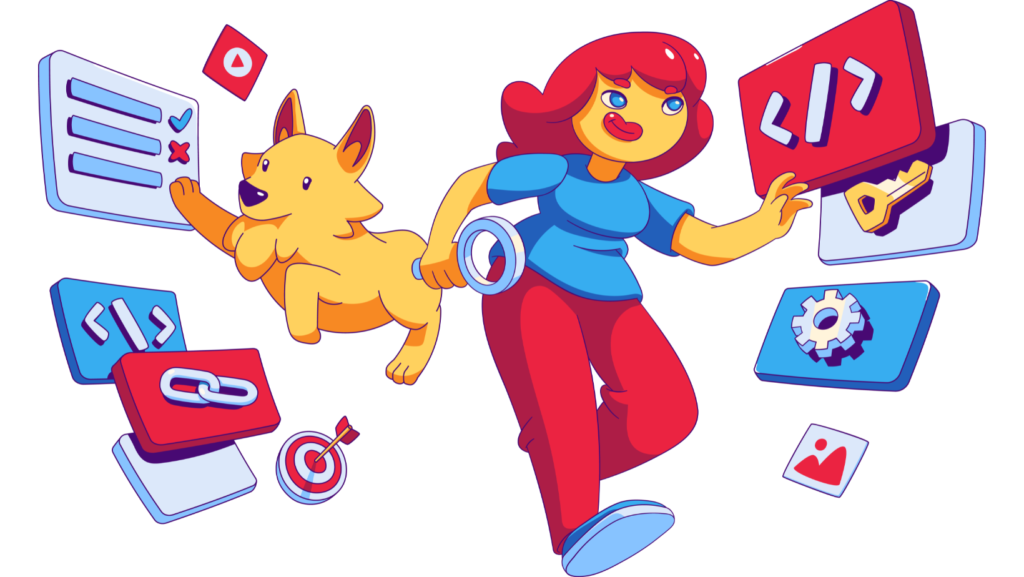